NodeJS xlsx Excel
최근 회사에서 OTA(Over The Air) 차량 업데이트 관련 업무를 진행하였다.
업데이트는 Button 클릭 시 HTTP Request를 통해 이루어지는 방식이다.
Button 클릭 시 총 5번의 Request가 일어나며 Polling이 포함되어 있고 소요 시간은 최대 1분이다.
Button을 클릭하여 1분 동안 대기 후 업데이트 결과를 확인하여 Excel 파일에 기록해야 했다.
설치
npm i xlsx
Parsing 할 xlsx 파일(예시)에 내용은 다음과 같다.
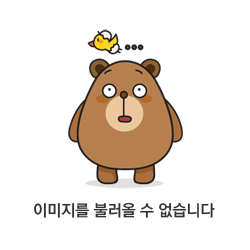
xlsx.readFile(Path, ParsingOption)을 통해 Parsing 할 xlsx 파일 전체를 읽은 후
Sheet 메서드를 사용해 특정 시트만 읽는다.
Parsing 된 시트는 Sheet_to_json을 통해 JSON 형식의 객체로 변환시킨다.
import xlsx from "xlsx";
import path from "path";
const __dirname = path.resolve();
const work = xlsx.readFile(__dirname + "/data.xlsx");
const workSheet = work.Sheets.list;
const records = xlsx.utils.sheet_to_json(workSheet);
JSON 형식 등 다양한 형식으로 변환이 가능하다.
sheet_to_json
sheet_to_txt
sheet_to_html
sheet_to_csv
sheet_to_formulae
변환된 JSON 객체는 배열 안 객체로 들어있으며
for of와 entries를 통해 반복문을 실행하면 다음과 같은 형태로 출력된다.
const records = xlsx.utils.sheet_to_json(workSheet);
for (const [i, e] of records.entries()) {
console.log(i, e);
}
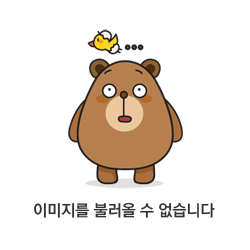
다음은 Write를 해야 한다. 새 파일 생성 후 다음 코드를 작성한다.
해당 코드는 참고 자료를 통해 알게 되었으며 Excel Sheet에 Write를 해주는데 정확한 작동 원리는 몰라도 된다.
import xlsx from "xlsx";
const rangeAddCell = (range, cell) => {
var rng = xlsx.utils.decode_range(range);
var c = typeof cell === 'string' ? xlsx.utils.decode_cell(cell) : cell;
if (rng.s.r > c.r) rng.s.r = c.r;
if (rng.s.c > c.c) rng.s.c = c.c;
if (rng.e.r < c.r) rng.e.r = c.r;
if (rng.e.c < c.c) rng.e.c = c.c;
return xlsx.utils.encode_range(rng);
}
export const addToSheet = (sheet, cell, type, raw) => {
sheet['!ref'] = rangeAddCell(sheet['!ref'], cell);
sheet[cell] = { t: type, v: raw };
};
다음은 Idx를 기준으로 짝수와 홀수를 판단해 결과를 입력하는 코드다.
실행 시 addToSheet("Sheet", "Cell", "자료형", "입력 값")을 통해 Column(결과)을 입력 후 조건에 따라 Cell 별로 값을 입력한다.
최종적으로 xlsx.writeFile("xlsx 파일", Path)로 결과물을 뽑아낸다.
(writeFile을 실행시키지 않으면 결과물이 출력되지 않는다)
import xlsx from "xlsx";
import path from "path";
import { addToSheet } from "./utils/add.to.sheet.js";
const __dirname = path.resolve();
const work = xlsx.readFile(__dirname + "/data.xlsx");
const workSheet = work.Sheets.list;
const records = xlsx.utils.sheet_to_json(workSheet);
for (const [i, e] of records.entries()) {
addToSheet(workSheet, "C1", "s", "결과");
const condition = e["Idx"];
const currentCell = `C${i + 2}`;
if (condition % 2 === 0) addToSheet(workSheet, currentCell, "s", "even");
else addToSheet(workSheet, currentCell, "s", "odd");
xlsx.writeFile(work, __dirname + "/result.xlsx");
}
다음은 writeFile을 통해 뽑힌 파일(result.xlsx)이며 각자 상황에 맞게 적절히 사용하면 될 거 같다.
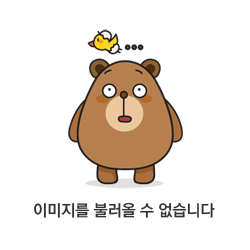
참고 자료
https://www.npmjs.com/package/xlsx
https://velog.io/@hahaha/Node.js-xlsx-%EB%AA%A8%EB%93%88
'서버 > Node' 카테고리의 다른 글
NodeJS 작동 원리 (0) | 2022.07.25 |
---|---|
NodeJS (0) | 2022.07.23 |
NodeJS Crawling Puppeteer (0) | 2022.04.18 |
NodeJS axios cheerio xlsx (0) | 2022.04.18 |
NodeJS dotenv (0) | 2022.01.20 |